How to use Google Calendar API with React JS
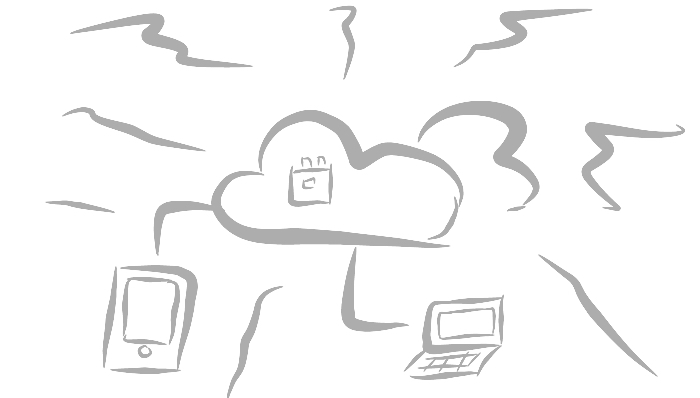
First goto Google Cloud console and get your Google API Key and head over to Google Calendar and grab your Calendar ID from settings, and also make sure the calendar is enabled to be publicly accessible.
First include the Google API script at the bottom of your body tag in index.html
https://apis.google.com/js/api.js
Create a new React component and define a state variable named events as an array.
class App extends React.Component{
constructor(props) {
super(props)
this.state = {
events: []
}
}
...
}
And then create a function named getEvents() which be can called during ComponentDidMount lifecycle of React JS.
componentDidMount = () => {
this.getEvents();
};
Let’s define our getEvent function, which make call to Google Calendar API and update the state with response.
getEvents(){
let that = this;
function start() {
gapi.client.init({
'apiKey': GOOGLE_API_KEY
}).then(function() {
return gapi.client.request({
'path': `https://www.googleapis.com/calendar/v3/calendars/${CALENDAR_ID}/events`,
})
}).then( (response) => {
let events = response.result.items
that.setState({
events
}, ()=>{
console.log(that.state.events);
})
}, function(reason) {
console.log(reason);
});
}
gapi.load('client', start)
}
The function gapi.load
is used to load gapi libraries. It needs two params. First one for libraries and second one is a callback function which can be triggered once the requested libraries are loaded.
We have given start as a callback function, which will initializes the JavaScript client with API key.
Once the client is initialised, we will call the request method which will take the Google Calendar API’s rest URL.
https://www.googleapis.com/calendar/v3/calendars/${CALENDAR_ID}/events
The above API endpoint will fetch all the events from calendar.
Once the request promise is resolved we will get the list of events as response. Then we will call setState method of React to store data to the app state.
Then we can render the list of events inside our app using code below:
...
render(){
return(
{this.state.events.map(function(event){
return(
{event.summary} {event.start.dateTime} - {event.end.dateTime}
)
});
)
}
...
That’s it you have successfully integrated Google Calendar API with React JS.